Last Updated on July 19, 2024 by Team Experts
File upload is an essential component of the majority of today’s modern web apps and websites. For instance, social media platforms like Facebook and Instagram must upload image and video files to servers from web clients. Similarly, some apps like Coursera require document upload.
Did you know you can do that quickly and easily with Angular file upload? However, you must ensure that a file upload with Angular is secure and device compatible.
Attackers can insert viruses into your website when you don’t secure file upload and compromise the server. You can also add a drag-and-drop feature to your Angular file upload to enhance your website’s usability.
This guide will discuss everything you need to learn about file upload with Angular.
What Is Angular?
Angular is a popular JavaScript framework that allows you to create scalable web apps using component-based architecture. It is essentially a TypeScript-based framework developed and maintained by Google.
A popular feature of Angular is that it allows you to reuse the code. Additionally, it supports two-way data binding, allowing your app’s components to share data.
Another impressive feature of Angular is route-level code splitting. This feature splits the functionality of each route of your angular app into several separate/individual chunks to reduce your app’s load time.
Today, thousands of businesses worldwide use Angular to create high-performance single-page applications that can be used to upload files. Angular is, in fact, one of the easiest and fastest file uploaders.
It supports a declarative UI (User Interface), allowing developers to create a UI with the data received. It also has several useful built-in features, like routing, state management, HTTP services, and dependency injection.
How To Create A File Upload With Angular?
Here is how you can create a file upload in Angular:
Creating An Angular App
First, create an Angular application using the code below:
ng new angular-file-upload |
Entering cd Command
Next, enter the following cd command:
cd src/app/ |
Create A New File Upload Component
ng g c file-upload/ |
Next, open an src/folder and edit the component.
Creating A Service For The Component
Now create a service for the file upload component using the command below:
ng g s file-upload/ |
Open the src/app/file upload folder and edit.
Finally, run the command below to serve on localhost:
ng serve |
Why Is It Important To Build A UI Of A File Upload Component?
When you use a plain HTML input of type file for file uploads, it’s challenging to style it properly. For this reason, it’s best to hide that from the end-user and build a new, attractive file upload UI that accepts the file input.
This UI can be a drag-and-drop zone, a dialog, or a button:
What Are Different Ways To Achieve File Upload With Angular?
There are several components within Angular that you can use to upload files manually. Depending on how you intend to use the file uploads, you can use these components.
Here are the most common components for achieving file upload with Angular:
FormData
FormData, as the name suggests, is used to deal with form data. It allows developers to store key-value pairs representing the form fields and their values.
This feature enables you to send file uploads to REST API endpoints through the XMLHttpRequest interface or HTTP client libraries. It’s important to note that FormData isn’t an Angular-specific component.
HttpClientModule
HttpClientModule is a module containing an API. Developers can use it to send and retrieve data for their apps from HTTP servers. Angular provides this service module.
Reactive Forms
Reactive forms, also called model-driven forms, consist of multiple validator functions for different use cases. These forms allow you to:
- Ensure data integrity between changes
- Validate form values
- Change the value of a control programmatically
- Use more than one control in a form group
- Modify controls dynamically in forms
These forms don’t mutate the form state. This means every time a change is made to the form, the existing data model isn’t updated. Rather, the FormControl instance will return a new data model.
Additionally, these forms are reusable and quite helpful with large-scale forms. To create Reactive forms, you need to import ‘ReactiveFormsModule.’
How To Upload Files With Angular?
To send files to the server, you need to send an HTTP POST multipart request where the Content-Type header should probably be set to multipart/form-data.
Here is how you can successfully upload files with Angular:
Importing The HttpModule
First, import the HttpModule in app.module.ts and then follow the steps below:
@NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, AppRoutingModule, FormsModule, // required for input file change detection ReactiveFormsModule, HttpClientModule, // this is required for the actual http request ], providers: [], bootstrap: [AppComponent] }) export class AppModule { } |
Creating Upload Button
Next, make the upload button and change event in app.component.html
<div class=”container”> <div class=”row mt-5″> <div class=”col-md-4″> <div class=”mb-3″> <label for=”formFile” class=”form-label”>Upload file example</label> <input (change)=”this.onFilechange($event)” class=”form-control” type=”file” id=”formFile”> <button (click)=”this.upload()” type=”button” class=”btn btn-primary mt-3″>Upload</button> </div> </div> </div> </div> |
To get the file content, you need to pass the event to the ts file.
Sending The Selected File To The Upload Service
One of the best practices to consider while working with Angular is to offload network-related operations to another service. For this reason, we simply wait for the upload button to click in the app component, and then send the file to the upload service in app.component.ts.
import { UploadService } from ‘./upload.service’; import { Component } from ‘@angular/core’; @Component({ selector: ‘app-root’, templateUrl: ‘./app.component.html’, styleUrls: [‘./app.component.css’] }) export class AppComponent { file: File = null; constructor( private uploadService: UploadService ){ } onFilechange(event: any) { console.log(event.target.files[0]) this.file = event.target.files[0] } upload() { if (this.file) { this.uploadService.uploadfile(this.file).subscribe(resp => { alert(“Uploaded”) }) } else { alert(“Please select a file first”) } } } |
Creating Service Controllers For Network Calls
The code below shows how to set service for network calls using upload.service.ts. The service essentially takes care of the upload process.
import { Upload Service } from ‘./upload. Service’; import { Component } from ‘@angular/core’; @Component ({ selector: ‘app-root’, template URL: ‘./app.component.html’, styleUrls: [‘./app.component.css’] }) export class AppComponent { file: File = null; constructor( private uploadService: UploadService ){ } onFilechange(event: any) { console.log(event.target.files[0]) this.file = event.target.files[0] } upload() { if (this.file) { this.uploadService.uploadfile(this.file).subscribe(resp => { alert(“Uploaded”) }) } else { alert(“Please select a file first”) } } } |
What Is Filestack, Can It Do Angular File Upload?
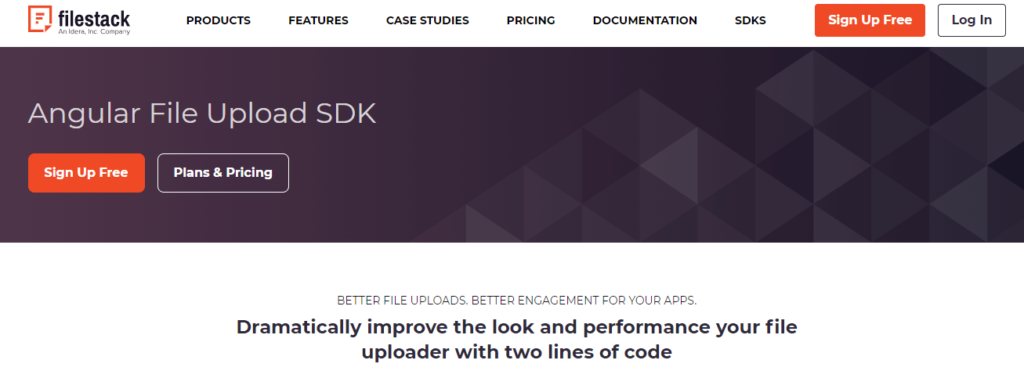
Filestack is a fast, secure, and reliable file upload API that allows you to enable user-friendly file uploads in your apps. As a result, it helps you improve user engagement with your apps.
Here are the key features of Filestack:
Angular File Upload SDK: Filestack comes with an Angular file upload SDK that enables efficient and secure file upload with Angular. It also comes with JavaScript, PHP, and Python SDKs.
Drag-and-Drop Feature: You can use Filestack to allow users to drag-and-drop or copy and paste files, such as videos, images, and audio files.
Multiple File Uploads: With Filestack, users can easily upload multiple files simultaneously.
Progress Bar: With the progress bar, you can show end-users that their files are on the way.
Client-Side Crop: With Filestack, end-users can easily crop files before sending them to your web app or website.
Also read: Angular Authentication for Secure Web Applications